How to do bulk updates in MongoDB
Bulk updating in MongoDB can be done using the update and updateMany methods.
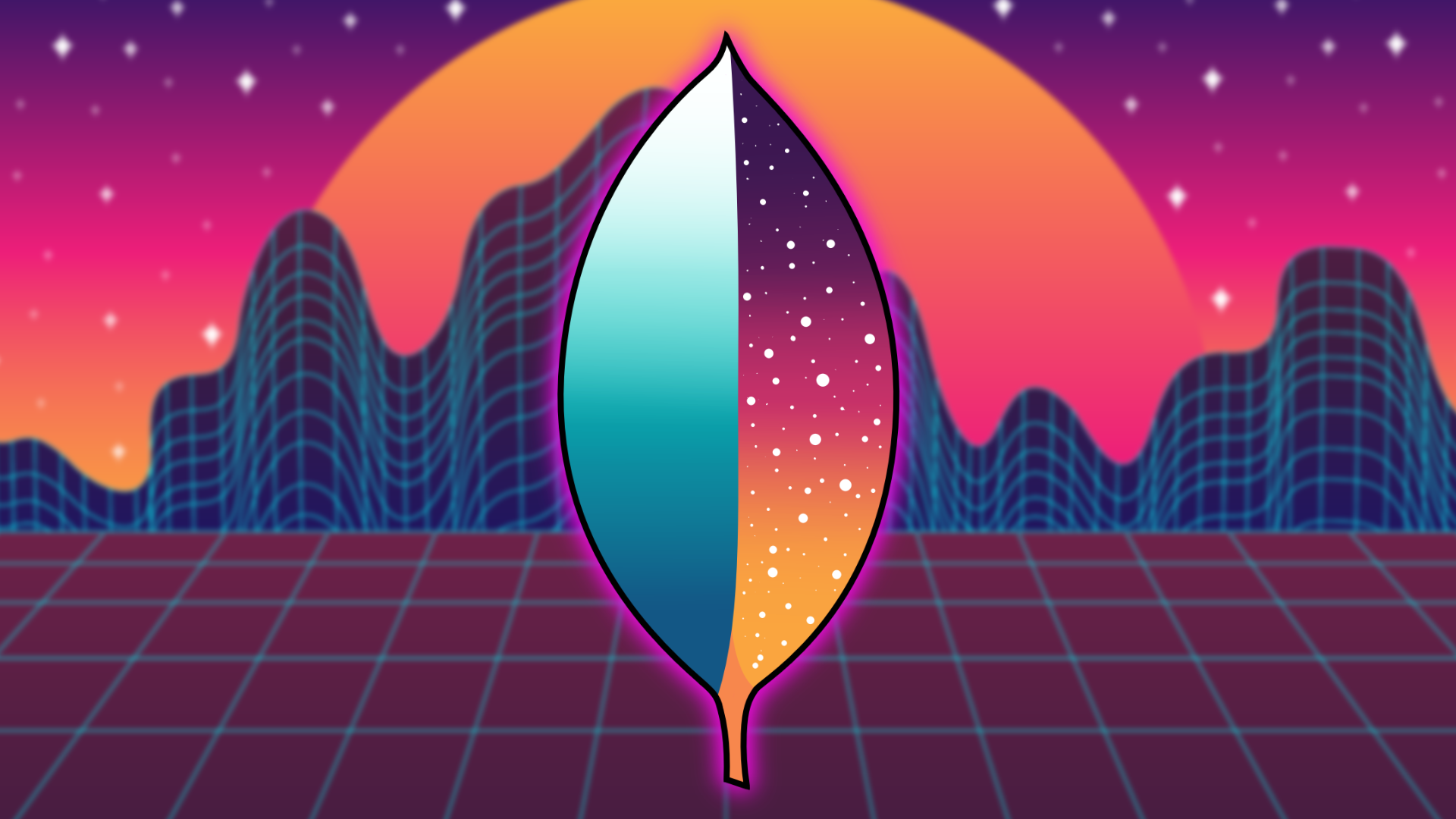
For a full overview of MongoDB and all my posts on it, check out my overview.
MongoDB provides several ways to update specifically one document and bulk updating is done in almost the same way.
Using the following data:
db.podcasts.insertMany([
{
"name": "Tech Over Tea",
"episodeName": "#75 Welcome Our Hacker Neko Waifu | Cyan Nyan",
"dateAired": ISODate("2021-08-02"),
"listenedTo": true,
},
{
"name": "Tech Over Tea",
"episodeName": "Neckbeards Anonymous - Tech Over Tea #20 - feat Donald Feury",
"dateAired": ISODate("2020-07-13"),
"listenedTo": true
},
{
"name": "Tech Over Tea",
"episodeName": "#34 The Return Of The Clones - feat Bryan Jenks",
"dateAired": ISODate("2020-10-19"),
"listenedTo": false
},
{
"name": "Cinemassacre Podcast",
"episodeName": "AVGN Fan Q&A, Starting a Band, and the Last Year - Cinemassacre Podcast",
"dateAired": ISODate("2021-08-10"),
"listenedTo": true
}
])
Let's update all the "Tech Over Tea" podcasts entry to be marked as listened to using update
. In order to update multiple documents using update
, we have to pass in an optional argument to tell MongoDB to do that.
db.podcasts.update(
{name: "Tech Over Tea"},
{$set: { listenedTo: true} },
{multi: true}
)
We can achieve the same result using updateMany
.
db.podcasts.updateMany(
{name: "Tech Over Tea"},
{$set: { listenedTo: true} }
)
Did you find this information useful? If so, consider heading over to my donation page and drop me some support.
Want to ask a question or just chat? Contact me here